8. Webhook
A webhook(also called a web callback) is a way for an application to provide real-time information. GHTK uses webhook to send notifications to partners upon order status changes. Every partner needs to provide GHTK a callback URL endpoint to receive instant updates upon order status changes.
E.g: callback URL
https://partner_example.com/updateShipment?hash=XXX
Example: an order with label ID: "S1.A1.17373471" (partner ID: "1234567") has been updated "successfully delivered".
GHTK system will send to partner’s callback link as below:
{
"partner_id" : "1234567",
"label_id": "S1.A1.17373471",
"status_id": 5,
"action_time": "2016-11-02T12:18:39+07:00",
"reason_code": "",
"reason": "",
"weight": 2.4,
"fee": 15000,
"pick_money": 100000,
"return_part_package": 0
}
Suppose partner’s callback link is https://partner_example.com/updateShipment?hash=XXX
Request sent from GHTK’s server to partner’s system:
- HTTP
- CURL
- PHP
POST /updateShipment?hash=XXX HTTP/1.1
Host: doitac.example.com
Content-type: application/x-www-form-urlencoded
label_id=S1.A1.17373471&partner_id=1234567&action_time=2016-11-02T12:18:39+07:00&status_id=5&reason_code=&reason=&weight=2.4&fee=1500&return_part_package=0
curl -X POST -H "Content-Type: application/x-www-form-urlencoded" \
-d 'label_id=S1.A1.17373471&partner_id=1234567&action_time=2016-11-02T12:18:39+07:00&status_id=5&reason_code=&reason=&weight=2.4&fee=1500&return_part_package=0' \
"https://doitac.example.com/updateShipment?hash=XXX"
<?php
$data = array(
'label_id' => 'S1.A1.17373471',
'partner_id' => 1234567,
'action_time' => '2016-11-02T12:18:39+07:00',
'status_id' =>5,
'reason_code'=> '',
'reason'=> '',
'weight'=> 2.4,
'fee'=> 1500,
'return_part_package'=> 0
);
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://doitac.example.com/updateShipment?hash=XXX",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_POSTFIELDS => $data,
));
$response = curl_exec($curl);
curl_close($curl);
echo 'Response: ' . $response;
?>
GHTK uses HTTP Status codes in responses to determine whether updates have been successfully sent to partners.
HTTP/1.1 200 OK
If the response is null or different from 200, GHTK will resend the request
HTTP/1.1 500 Internal Server Error
Input parameters
Parameter | Datatype | Description |
---|---|---|
label_id | String | GHTK’s reference ID (order.label) |
partner_id | String | Partner’s reference ID |
status_id | Integer | Status code (refer to table below for list of status code) |
action_time | String | represents time of updating order status .Format: ISO 8601 |
reason_code | Integer | Reason code for update |
reason | String | Reason in text |
weight | Float | Package weight in kilogram |
fee | Integer | Shipping fee (in VND) |
return_part_package | Integer | To indicate if the order is a partial shipment. "1" if it is partial shipment |
Order Status Details:
Status | Description |
---|---|
-1 | Canceled order |
1 | Not Confirmed: order submitted to GHTK’s system is awaiting to be validated and confirmed by the distributor. If shop is configured Automatic Confirmation and all the order’s information is valid, this status is "Confirmed" in default. |
2 | Confirmed: The order is successfully submitted and a carrier will be assigned to pick up the order |
3 | Picked/In warehouse: Carrier picked the order and put it in warehouse |
4 | Delivering: carrier is on the way to deliver the order |
5 | Delivered: order is delivered successfully |
6 | Reconciled (In case of successful Delivery. Prior status is "5: Delivered") |
7 | Failed to Pick Up (over 3 picking-up attempts or shop requested to cancel the order before it is picked up) |
8 | Delay Picking Up |
9 | Failed to Deliver (over 3 delivering attempts). The return process will be triggered then |
10 | Delay Delivering |
11 | Reconciled (in case of Failed to deliver. Prior stage is "9: Failed to Deliver") |
12 | Picking Up: carrier is on the way to pick up the order |
13 | Compensation order |
20 | Returning: carrier attemtps maximum 3 times to return |
21 | Returned: Package order is returned to shop |
123 | Carrier reports order is picked up |
127 | Carrier reports order is failed to pick up |
128 | Carrier reports delay picking up |
45 | Carrier reports order is delivered |
49 | Carrier reports order is failed to deliver |
410 | Carrier reports delay delivering |
Note:123, 127, 128, 45, 49, 410 are internal processing stages for notification only and not returned as order status in this API.
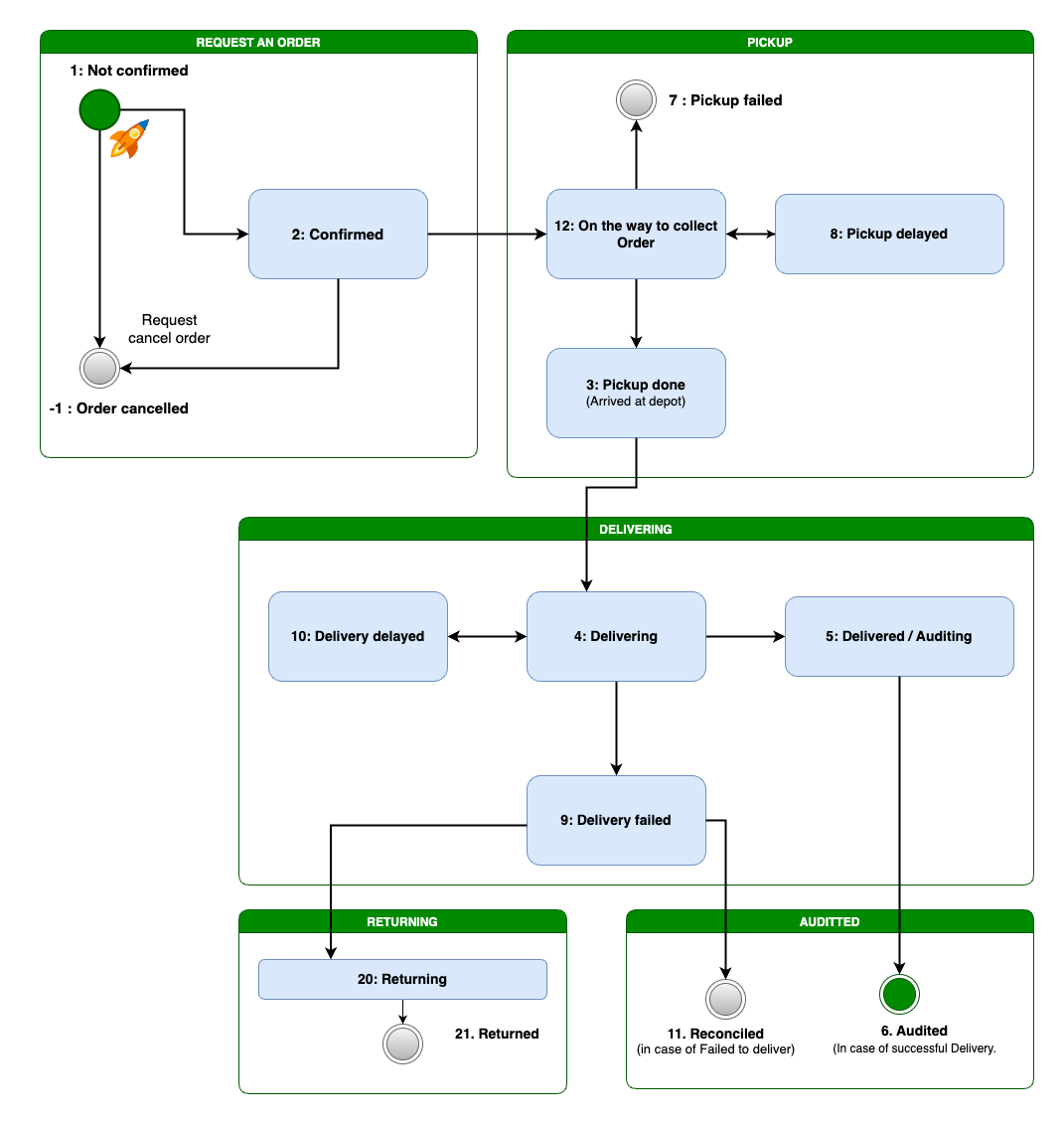
Reason code for Delay Picking Up (status_id=8):
reason_code | Description |
---|---|
100 | Shop requests to delay picking up until the next shift |
101 | Unable to contact shop owners |
102 | Package order is not ready for picking up |
103 | Shop changes pick-up address |
104 | Shop changes the date of picking-up |
105 | Carrier is overloaded |
106 | Objective factors (e.g Weather) |
107 | Others |
Reason code for Failed to Pick Up (status_id=7):
reason_code | Description |
---|---|
110 | Pick up address is not supported |
111 | Product is not allowed to deliver (e.g prohibited products) |
112 | Shop requests to cancel order |
113 | Over 3 pick-up attempts |
114 | Others |
115 | Partner requests to cancel order via API |
Reason code for Delay Delivering (status_id=10):
reason_code | Description |
---|---|
120 | Carrier is overloaded |
121 | Customer requests to delay until the next shift |
122 | Unable to contact customer |
123 | Customer requests to receive at another time |
124 | Customer changes delivery address |
125 | Wrong address, shop needs to double check |
126 | Objective factors (e.g weather conditions) |
127 | Others |
128 | Shop changes delivery time |
129 | Package is not found |
1200 | Wrong contact number, shop needs to double check |
Reason code for Failed to Deliver (status_id=9):
reason_code | Description |
---|---|
130 | Customer refuses to recieve the products. |
131 | Unable to contact over 3 times |
132 | Customer requests to delay over 3 times |
133 | Shop requests to cancel order |
134 | Others |
135 | Partner requests to cancel order via API |
Reason code for Delay Returning:
reason_code | Description |
---|---|
140 | Shop requests to delay until the next shift |
141 | Unable to contact shop owners |
142 | Shop is not at returning address |
143 | Shop requests to receive returning order at another time |
144 | Others |